Leveraging benchstat Projections in Go Benchmark Analysis!
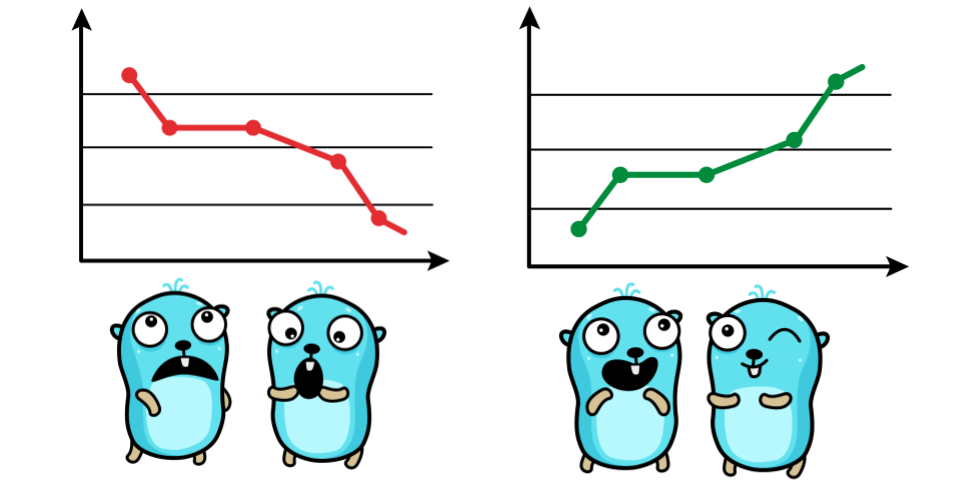
Go’s built-in micro-benchmarking framework
is extremely useful and widely known. Sill, not many developers are aware of the additional, yet essential, benchstat
tool
allowing clear comparisons of Go A/B benchmark results across multiple runs. In 2023, benchstat
received a complete overhaul making it even more powerful: projections, filtering and groupings were introduced allowing robust comparisons across any dimension, defined by your sub-benchmarks (aka “cases”), if you follow a certain naming format
.
In this post, we will get you familiar with the benchstat
tool and some Go benchmarking best practices. If you have read an older version of my “Efficient Go” book
, this article will get you updated on the recent benchstat
features.
So… get that terminal warmed up by installing benchstat
with the go install golang.org/x/perf/cmd/benchstat@latest
command and let’s go!
Old-school Flow: Comparing Efficiency Across Versions
Let’s start by explaining the most popular iterative benchmarking flow, where we run the same benchmark on multiple versions of your code. Generally, the flow works by:
-
Creating the benchmark test code
Creating a benchmark is as simple as creating a
func BenchmarkFoo(b *testing.B)
testing function in youbar_test.go
file. Inside, you can optionally use multipleb.Run(...)
cases to benchmark different cases for similar functionality. I wrote about Go benchmarking extensively in my “Efficient Go” book (Chapter 8) , but there are also free resources e.g. surprisingly up-to-date 10y old good Dave’s Cheney blog post . -
Running the benchmark for the version A of your code
To run the
BenchmarkFoo
testing function quickly, for the default 1s time, you can use thego test -bench BenchmarkFoo
command. This is good for testing things out, but typically we use more advanced options like (essential!) multiple runs (-count
), CPU limits (-cpu
), profiling (-memprofile
) and more. In my book I recommend pairing it withtee
so you stream the output to bothstdout
and file for future reference e.g.v1.txt
:export bench=v1 && go test \ -run '^$' -bench '^BenchmarkFoo' \ -benchtime 5s -count 6 -cpu 2 -benchmem -timeout 999m \ | tee ${bench}.txt
The resulting output contains absolute results (allocations, latency, custom metrics) from that benchmark run(s).
-
Optimize the code you are benchmarking
You can then
git commit
whatever you had (just to not get lost!), and change the code you are benchmarking (e.g. in an attempt to optimize it based on previously gathered profiles). -
Running the benchmark for the version B of your code
Now it’s time to execute the same benchmark to see if your optimization is actually better or worse, while changing the output name to get lost e.g. in the
v2.txt
file. -
Analyze the A/B benchmark results
Once we have old and new (A and B) results, it’s time to use the
benchstat
tool ! Runbenchstat base=v1.txt new=v2.txt
to compare two versions. You will still see the absolute latency, allocations (and any custom metrics you reported) numbers, but what’s more important–the relative percentages of improvements/regressions for those, and the probability of noise.
After those steps, you likely know if new changes improved CPU latency or memory consumption or made it worse, or if you have to repeat (or fix!) the benchmark due to noise. Let’s go through a specific example!
Example
To showcase this, I wrote a quick example benchmark ported from the real microbenchmark I did when preparing for the PromCon talk about Remote Write 2.0 .
The main benchmark goal is to compare the encoding efficiency of the Remote Write 1.0 protocol to the 2.0 version for different sample sizes, ideally across different compressions and two different Go protobuf encoders (marshallers). If we use “different versions” flow it may look like this:
|
In this flow, one could comment things out or in, or change directly and repeat the benchmark while changing the output file in the handy export bench=<file-name> ...
CLI command.
After old (v1) and new (v2) results are generated (in this example v1
means Remote Write 1.0
proto message and v2
means 2.0), we can use benchstat
to compare it:
|
The above tells us that Remote Write 2.0 is both smaller on the wire and uses less CPU and Memory to encode (and compress) with zstd
compression.
Pros & Cons
While this traditional approach to Go micro benchmarking (modifying code and rerunning benchmarks) is simple for interactive, quick tests, it also has significant drawbacks:
-
Difficult to track changes: It’s easy to lost track of what exactly you benchmark, especially if you notice your current optimizations are not helping, and you need to revert to some previous state.
-
Accidental benchmark changes: Unintentional modifications to the benchmark code itself can lead to unreliable comparisons and are hard to notice in this flow.
-
Limited collaboration: Sharing and replicating benchmarks becomes challenging. This is a big blocker for bigger projects, where reviews need to ensure the reliability of the author’s benchmark and claimed results.
No one assumes the wrong intention, but it’s extremely easy to make a little mistake in benchmarking, so replicating benchmarks by multiple people is highly recommended before making decisions. Recently more ways to do this on CI emerged too e.g. github-action job for benchmarks which helps a bit with that, but not with the small iterations you do on your own.
-
Environmental inconsistencies: Micro-benchmarks are NOT about the absolute values, but the relative difference of resource use and latency across benchmark runs. This is because we want to run them locally, for a fast development feedback loop and low risk, instead of the actual production environment with all the production dependencies. However, even relative numbers can be unreliable e.g. if you benchmark different code version across different hardware or on the same hardware, but in a different conditions (e.g. different browser tabs opened during benchmarks!).
As a result, the longer you work on your next version, the more unreliable your benchmark process is in practice. This can be mitigated by going back (e.g. in git) to the old version, running a benchmark, then going to the new code, doing it again to minimize the “time” gap between benchmark runs. The other interesting mitigation I saw e.g. in Dave Cheney’s flow is to compile binaries with benchmark tests and keep them somewhere, well-described, so you can always execute benchmarks one after another. Both mitigations are a bit painful in practice.
-
Complex comparisons: The above issues are even bigger if you want to benchmark your code across different cases like we did in our example.
These limitations are why I am excited to share an alternative flow, enabled with the new benchstat
changes!
Newly Enabled Flow: Comparing Efficiency Across Cases
While I was always enjoying benchstat
I did miss an important feature–instead of comparing the benchmark results stored in different files, I wanted to compare the runs across b.Run(...)
sub-benchmarks/cases. Such a comparison flow was becoming more handy (at least for my mental model), the more I was doing benchmarks. I even initially planned to contribute/write another tool for that.
Gladly I didn’t, because in January 2023, benchstat
was rewritten
. Austin Clements, with reviewers, added a new flexible filtering and control on what you want to compare with what. The rewrite also improved other things e.g. warnings insufficient -count
(number of benchmark runs) to detect and reject outliers and overall detection of non-matching results.
The idea behind this flow is simple–for reproducibility, reliability and clarity we try to capture the new and old “code” as different cases. Anyone can run this benchmark once and produce a single result file. Finally, anyone can use a new benchstat
projection and filtering features to compare results from that one run, across different dimensions, on the fly.
One important detail to remember when making things work with the benchstat
projection feature, is that all cases should follow a proposed format
. Funny enough, this proposal was not changed since 2016, but only when writing this post I learned about this!
Specially, we need to ensure our b.Run(...)
sub benchmark case naming follow <case name>=<case value>
pair format. For example, to represent v1 and v2 protobuf versions, we could do proto=prometheus.WriteRequest
(which is the official unique package name for 1.0) and proto=io.prometheus.write.v2.Request
for 2.0.
Example
Let’s adapt the previous example into a new across_cases
example benchmark
.
Notice the obvious increase of the complexity for the benchmarking test and the strict syntax for sub-benchmark cases:
|
In this flow, we can just execute the export bench=allcases ...
CLI command once to produce allcases
file.
Now here is where the benchstat
projection magic comes in. We can use new syntax
to control what dimensions we want to compare across, what things we want to filter out or group by!
For example to produce similar output to the across version flow
we can use -filter "/compression:zstd /encoder:protobuf" -col /proto
parameters. We can also (optionally here) provide -row ".name /sample /compression /encoder"
to group by the remaining dimension explicitly:
|
|
We can also easily compare across compressions (switching to -col
and updating -row
), giving us amazing flexibility when new a question arises. We can also impact sorting order, no matter the original sorting in the raw allcases
result file, by re-ordering the keys in the -row
option:
|
👉🏽 Expand to see the output.
|
Pros & Cons
In general, this flow makes our benchmarking results a bit more reproducible and reliable by mitigating most of the downsides of across versions flow . However, it has some negative consequences too:
- Rerunning benchmarks with a large amount of cases takes significantly time (slower feedback loop!).
- It yields more complex benchmarking code, which makes it hard to iterate on, and spot places where you benchmark the testing code vs the portion of the code you wanted to.
- For continuous production use, it does not make sense to commit that benchmark with all cases, which are no longer being continued. It fits better to capture such a benchmark in some remote branch for future reference though.
Summary
To sum up, the new benchstat
version with projection feature enables local probing of OLAP
type of questions against your benchmarking results.
As with everything, the two presented flows (across versions and cases ) represent different trade-offs. None of those is objectively better or worse. I would recommend considering using both in a hybrid approach, depending on your goals.
In the mentioned example
, I found the across case
flow more beneficial for the real scenario Remote Write protocol benchmark. This is because the Prometheus code already supports multiple sample batches, and the implementations for different compressions and encoders were easily imported. We also have to support both the 1.0 and 2.0 protocol versions, so they already co-exist in the current codebase. Furthermore, given the protocol’s relative popularity I wanted to ensure everyone can reproduce the benchmarks and give feedback. All of those reasons make the case
flow a trivial choice here. However, from this point, if were iterating on optimizations to compression or protocol, I would likely follow versions
flow a bit.
Hopefully, at this point, you know what flow to use for what benchmarking needs in your engineering adventures! You are also welcome to check other useful benchstat
options e.g. I recently used the -format csv
option to get my comparisons into Google Sheets, so I can produce charts for my talk slides
. I also found asking Gemini
GenAI for chart rendering pretty useful and accurate, but old-good way still gives a bit more deterministic control on small details.
Finally, no matter what flow you will use, follow the proposed case syntax
. No harm in doing so, and you never know when somebody might want to use benchstat
projection for your benchmarks!
Last, but not least, I am here to learn too, so feel free to give feedback on what I could explain or do better! 🤗
Credits
As always, thanks to all reviewers (e.g. David, Manik!) and Maria Letta for the beautiful Gopher illustrations .
Comments